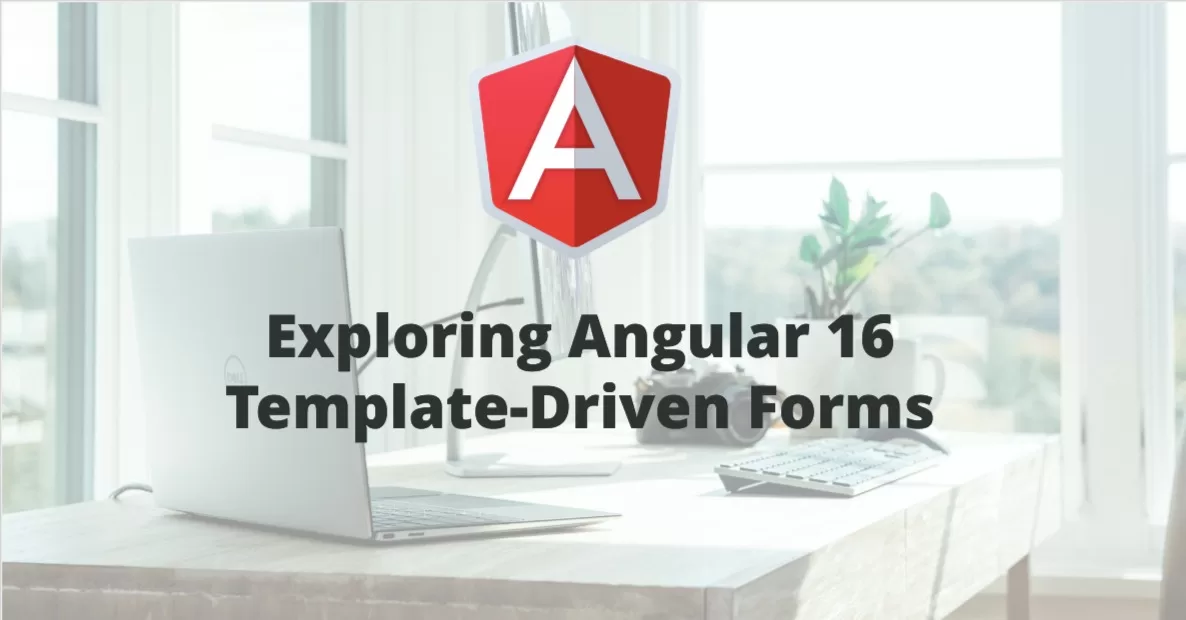
Angular 16 Template-Driven Forms: A Comprehensive Exploration
Contents
In the ever-evolving world of web development, staying up-to-date with the latest tools and frameworks is crucial. Angular has long been a favorite among developers for its powerful features and flexibility. Angular 16 Template-Driven Forms provide a straightforward way to handle form input and validation in your web applications. In this guide, we’ll explore how to create and work with Template-Driven Forms, which are a great choice for less complex forms. With the release of Angular 16, we have seen significant improvements in various aspects, including Template-Driven Forms. In this comprehensive guide, we will delve into Angular 16’s Template-Driven Forms, exploring their benefits and how to make the most of this feature.
Understanding Angular 16 Template-Driven Forms
Angular’s Template-Driven Forms provide an elegant and intuitive way to handle form inputs in your web applications. They offer a high level of abstraction, making it easier to create complex forms with minimal effort. Let’s break down the key components and features:
1. Form Structure
The first step in harnessing the power of Template-Driven Forms is to understand their structure. These forms are built directly into the HTML template and rely on directives to bind form controls to data models. This declarative approach simplifies form creation and management.
2. Two-Way Data Binding
Angular 16’s Template-Driven Forms leverage two-way data binding, allowing seamless synchronization between the UI and the underlying data model. Any changes made in the form fields are automatically reflected in the model and vice versa. This feature reduces the need for manual data manipulation.
3. Validation Made Easy
Validating user input is a crucial aspect of any web application. Angular 16 simplifies this process with built-in validators that can be easily added to form controls. Whether it’s checking for required fields, email formats, or custom validations, Template-Driven Forms have you covered.
4. Dynamic Form Generation
One of the standout features of Angular 16 Template-Driven Forms is the ability to dynamically generate form elements. You can add or remove form controls based on user interactions or business logic. This dynamic approach ensures that your forms remain flexible and adaptable to changing requirements.
To get started with Angular 16 Template-Driven Forms in Angular, follow these steps:
Import Forms Module
In your Angular 16 application, you need to import the FormsModule
from @angular/forms
in your app module. This module is essential for enabling Template-Driven Forms.
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
@NgModule({
imports: [
// ...
FormsModule
],
declarations: [
// ...
],
})
export class AppModule { }
Create Angular 16 Template-Driven Forms
In your component’s HTML template, you can create the form structure using HTML form elements. Bind form controls to template variables using ngModel
for two-way data binding. For example:
<form #myForm="ngForm" (ngSubmit)="onSubmit()">
<div class="form-group">
<label for="name">Name</label>
<input type="text" id="name" name="name" [(ngModel)]="formData.name" required minlength="2">
<div *ngIf="myForm.controls['name'].invalid && (myForm.controls['name'].dirty || myForm.controls['name'].touched)">
<div *ngIf="myForm.controls['name'].errors.required">Name is required.</div>
<div *ngIf="myForm.controls['name'].errors.minlength">Name should be at least 2 characters.</div>
</div>
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="email" id="email" name="email" [(ngModel)]="formData.email" required pattern="[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,4}$">
<div *ngIf="myForm.controls['email'].invalid && (myForm.controls['email'].dirty || myForm.controls['email'].touched)">
<div *ngIf="myForm.controls['email'].errors.required">Email is required.</div>
<div *ngIf="myForm.controls['email'].errors.pattern">Invalid email format.</div>
</div>
</div>
<div class="form-group">
<label for="phone">Phone</label>
<input type="tel" id="phone" name="phone" [(ngModel)]="formData.phone" required pattern="[0-9]{10}">
<div *ngIf="myForm.controls['phone'].invalid && (myForm.controls['phone'].dirty || myForm.controls['phone'].touched)">
<div *ngIf="myForm.controls['phone'].errors.required">Phone is required.</div>
<div *ngIf="myForm.controls['phone'].errors.pattern">Invalid phone number format (e.g., 1234567890).</div>
</div>
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" id="password" name="password" [(ngModel)]="formData.password" required>
<div *ngIf="myForm.controls['password'].invalid && (myForm.controls['password'].dirty || myForm.controls['password'].touched)">
<div *ngIf="myForm.controls['password'].errors.required">Password is required.</div>
</div>
</div>
<button type="submit" [disabled]="myForm.invalid">Submit</button>
</form>
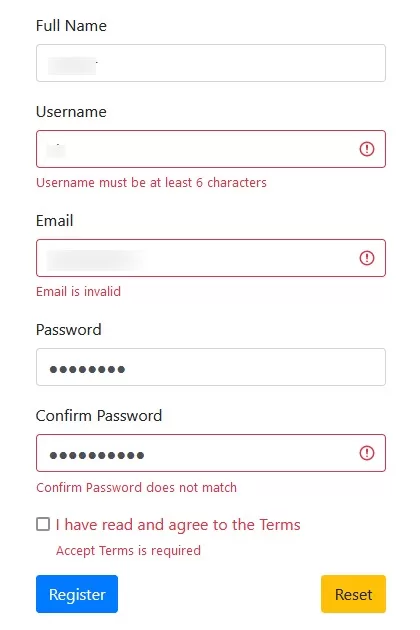
Handle Form Submission
In your component class, define the form data model and handle form submission logic.
import { Component } from '@angular/core';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
})
export class YourComponent {
formData = {
name: '',
email: '',
phone: '',
password: '',
};
onSubmit() {
if (this.myForm.valid) {
// Handle form submission here
}
}
}
This example demonstrates how to create an Angular 16 Template-Driven Forms with the specified field validations for name, email, phone, and password. Ensure you have the FormsModule imported, and you have the necessary HTML and component code in your Angular 16 Template-Driven Forms application.