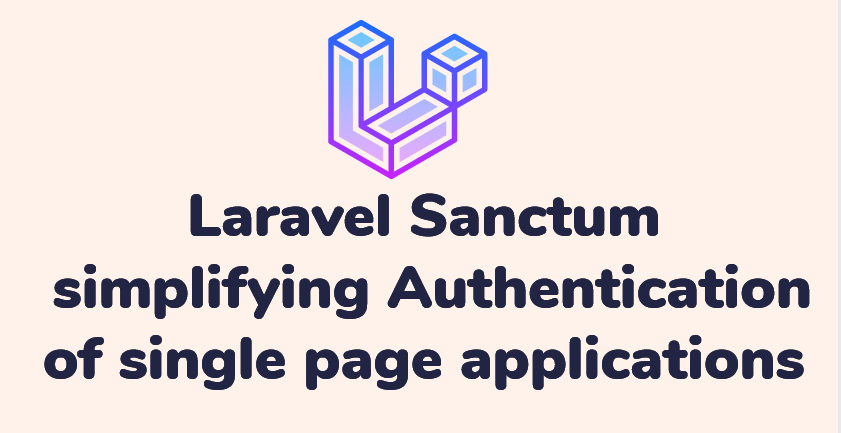
Discover Laravel Sanctum: Empower API Authentication
Contents
Ensuring that your Laravel application’s backend is secure is crucial in the modern API-driven environment. An appealing solution that provides a simple method for API permission and authentication is Laravel Sanctum. Laravel Sanctum offers a lightweight authentication solution for mobile apps, token-based APIs, and single page applications (SPAs). Every user of your application can create several API tokens for their account with Sanctum. These tokens might be given scopes or abilities that define what kinds of actions they can carry out. This article explores the main advantages of Sanctum, walks you through the installation procedure, and offers real-world examples to show how to use it.
Introduction to Laravel Sanctum
There are two distinct challenges that Laravel Sanctum aims to answer. Before we delve further into the library, let’s talk about each.
Tokens for APIs
First, if you want to give your users API tokens without the hassle of OAuth, you can utilize Sanctum, a straightforward package. Applications like GitHub and others that provide “personal access tokens” served as inspiration for this functionality. Consider an application where a user can generate an API token for their account on the “account settings” tab. Sanctum can be used to create and control those tokens. These tokens can be manually revoked at any moment by the user, however they normally have a very long expiration term (years).
Authentication of single page applications (SPAs)
Second, Sanctum was created to provide a straightforward method for authenticating single-page applications (SPAs) that have to talk to an API powered by Laravel. These SPAs can be in a different repository altogether, like an application built with Next.js or an SPA made with the Vue CLI, or they could be in the same repository as your Laravel application.
Sanctum uses no tokens at all for this feature. Sanctum, on the other hand, makes use of Laravel’s integrated cookie-based session authentication services. Usually, Sanctum does this by using the web authentication guard built into Laravel. This guards against the disclosure of the authentication credentials via XSS and offers the advantages of session authentication and CSRF protection.
Laravel Sanctum Process Cycle

Laravel Sanctum Advantages:
Sanctum simplifies API security by offering a number of significant benefits.
Simplified Authentication: Sanctum removes the difficulty associated with managing tokens by manually. Your Laravel application has an easy-to-use interface that lets users create and manage API tokens.
Token-Based Authentication: Secure API tokens are used by Sanctum for token-based authentication. In comparison to session-based authentication, these tokens provide a more effective and stateless method, improving scalability and speed.
Session-Based Authentication: You may authenticate users accessing your API from within the web application by utilizing Sanctum’s smooth integration with Laravel’s integrated session management. This makes the user experience more seamless.
CSRF Protection: By adding CSRF tokens to API requests, Sanctum protects against Cross-Site Request Forgery (CSRF) attacks. This extra security layer prevents nefarious attempts to access your application.
Installation of Laravel Sanctum
To install Laravel Sanctum, follow these steps:
1. Install the Sanctum package using Composer:
Before install sanctum package, Laravel must install to your system. For install Laravel
composer require laravel/sanctum
2. Run the following command to publish the Sanctum configuration and migration files:
php artisan vendor:publish --provider="LaravelSanctumSanctumServiceProvider"
3. Run Sanctum’s migrations to create the necessary tables:
php artisan migrate
Add Sanctum’s middleware to your API routes within the api
middleware group in your api.php
file:
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
Route::middleware(['auth:sanctum'])->get('/user', function (Request $request) {
return $request->user();
});
Laravel Sanctum: Real-time Example
Let’s imagine you are using Laravel and Vue.js to create a task management application. To protect your endpoints, API authentication must be implemented. To the rescue is Laravel Sanctum.
Prerequest
For run this demo install Laravel to your Laravel project.
Create a new authentication controller to handle user login and token generation:
php artisan make:controller AuthController
Implement login and token generation methods
within the AuthController
using Sanctum’s createToken
method:
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Illuminate\Validation\ValidationException;
use Laravel\Sanctum\PersonalAccessToken;
class AuthController extends Controller
{
public function login(Request $request)
{
$credentials = $request->validate([
'email' => 'required|email',
'password' => 'required',
]);
if (!Auth::attempt($credentials)) {
throw ValidationException::withMessages([
'email' => ['The provided credentials are incorrect.'],
]);
}
$user = User::where('email', $request->email)->first();
return $user->createToken('token-name')->plainTextToken;
}
}
Conclusion
Laravel Sanctum is a powerful and convenient authentication system for Laravel applications. Its simplicity, security, flexibility, and extensibility make it an ideal choice for building secure and scalable API-based applications. By following the installation steps outlined above, you can easily integrate Sanctum into your Laravel project and enjoy its numerous benefits.**Conclusion**
Laravel Sanctum is a powerful and convenient authentication system for Laravel applications. It provides a simple and secure way to authenticate users and manage API tokens. Sanctum is easy to install and configure, and it can be used with both web and API applications.