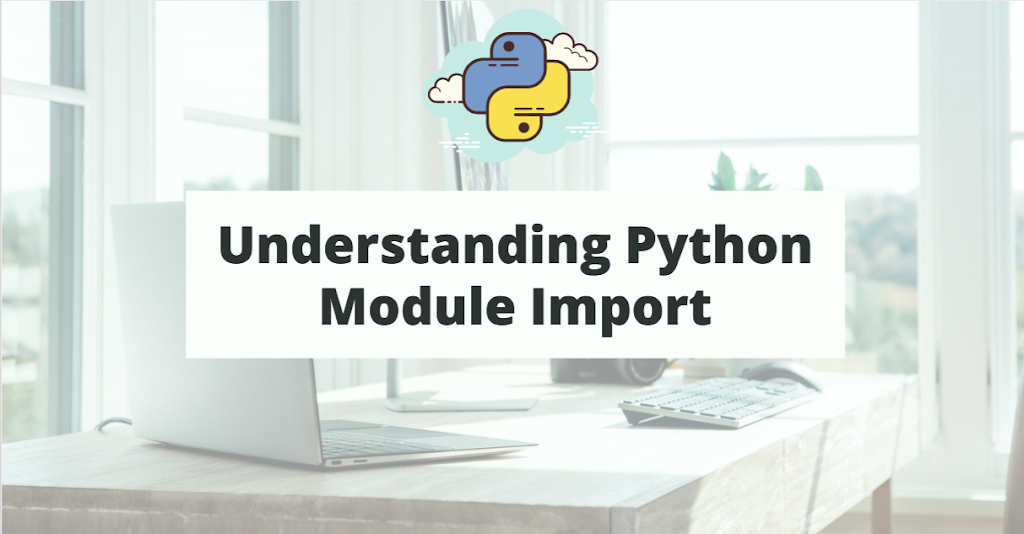
Python: Advantage of Module Import
Python is a powerful and versatile programming language that allows developers to modularize their code for better organization, reusability, and maintainability. One of the key concepts is Python module import, which allows you to use code from external modules or libraries in your own Python programs. In this comprehensive guide, we will explore various aspects of Python module import, including different ways to import modules, best practices, common issues, and troubleshooting tips.
Modules can contain variables, functions, classes, and other objects that can be accessed and used in your code. Python comes with a rich standard library that includes many built-in modules for common tasks, such as math, string manipulation, file I/O, and more. Additionally, there are numerous third-party modules available that extend Python’s functionality for specific tasks or domains, such as NumPy for numerical computing, Django for web development, and Pandas for data analysis.
To use a module in your Python program, you need to import it. There are several ways to import modules in Python, including:
import statement:
The most common way to import a module is by using the import statement, followed by the name of the module. For example, to import the math module for mathematical operations
you can use the following syntax:
import math
After importing the math module, you can use its functions and objects in your code by prefixing them with the module name, followed by a dot. For example:
x = math.sqrt(25)
from … import statement:
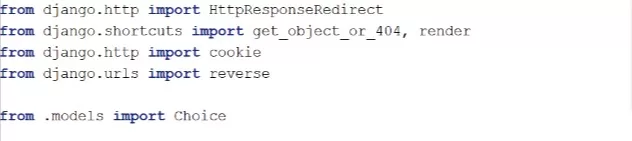
Another way to import modules is by using the from … import statement, which allows you to selectively import specific objects from a module into your code. For example, to import only the sqrt function from the math module, you can use the following syntax:
from math import sqrt
After using this statement, you can directly use the sqrt function in your code without prefixing it with the module name.
Python import … as … statement:
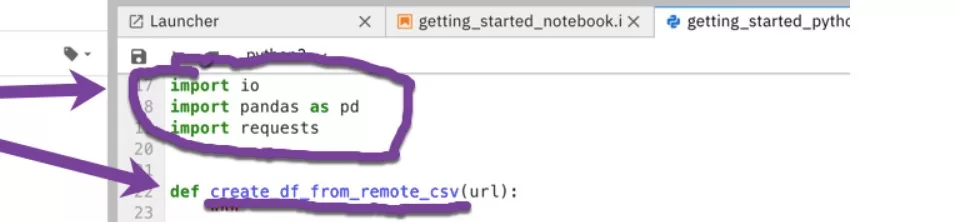
You can also use the import … as … statement to import a module with an alias, which allows you to use a shorter or more convenient name for the module in your code. For example, to import the panda’s module with an alias PD, you can use the following syntax:
import pandas as pd
After using this statement, you can use pd as a shorthand for the panda’s module in your code.
Once you have imported a module, you can use its objects and functions in your code as needed. However, it is important to be mindful of namespace clashes, where objects or functions from different modules have the same name, or naming conflicts with your own code. To avoid such issues, it is recommended to use explicit module prefixes or aliases and be aware of the scope and visibility of objects in your code.