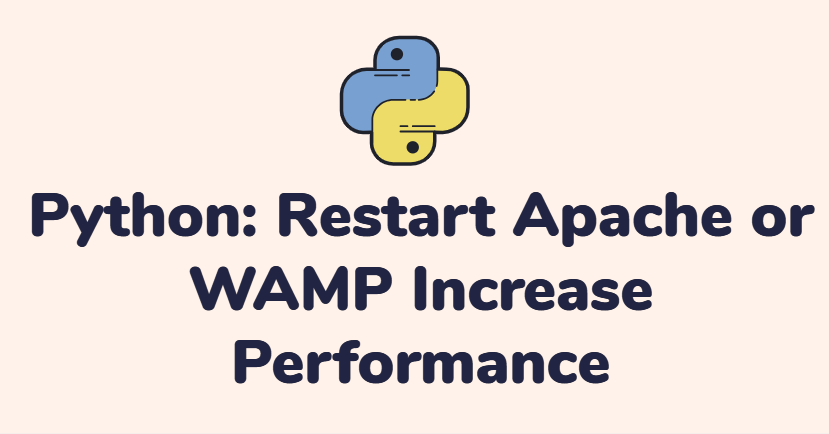
Python: Restart Apache or WAMP Increase Performance
Contents
There are a few different ways to programmatically restart Apache or WAMP depending on your operating system and programming language. Here are a few typical methods:
Command Line (Restart Apache)
If you are using WAMP on a Windows system, you can use the net
command to restart the Apache service. You can do this programmatically in a batch script or PowerShell script. If you don’t have WAMP server download WAMP and install it.
Batch Script Example:
net stop wampapache
net start wampapache
PowerShell Script Example:
Stop-Service -Name wampapache
Start-Service -Name wampapache
Command Line (Linux/macOS)
On Linux or macOS, you can use the systemctl
command to restart the Apache2.
sudo systemctl restart apache2

You can execute this command programmatically from a shell script or other scripting languages like Python.
Programming Language (Python)
You can use Python to programmatically restart Apache on both Windows and Linux. Here’s a Python script that uses the subprocess
module to execute the necessary commands:
import subprocess
# On Windows (using WAMP)
subprocess.run(["net", "stop", "wampapache"])
subprocess.run(["net", "start", "wampapache"])
# On Linux (using systemctl)
subprocess.run(["sudo", "systemctl", "restart", "apache2"])
Programming Language (PHP)
If you prefer to use PHP, you can also use the exec
or shell_exec
functions to execute system commands to restart Apache:
<?php
// On Windows (using WAMP)
exec("net stop wampapache");
exec("net start wampapache");
// On Linux (using systemctl)
exec("sudo systemctl restart apache2");
?>
When executing system commands programmatically, be careful as it can require elevated permissions, particularly on Linux systems. Make sure the script you’re running has the rights it needs to restart the Apache service. Furthermore, exercise caution when using these techniques and refrain from disclosing any security holes in your program.